JavaScript Browser, OS, and Device Detection
This script helps developers detect the browser type, operating system, and device type of users visiting their applications or websites. It incorporates robust functionality to handle common and uncommon browsers, supports detection of Brave browser, and includes OS and device type, detecting device is a mobile phone, tablet, or desktop computer.
In this article, we will explore several popular JavaScript libraries that make browser detection easier and more reliable.
Contents
What is Browser Detection?
Browser detection is the process of identifying the browser type, version, and features of a user's web browser. This can be done using various methods, including user agent detection, feature detection, and browser-specific APIs.
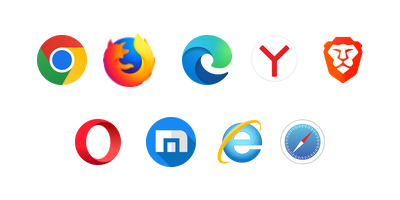
Browser Detection Code:
Here is the script used to detect browsers, operating systems, and devices. You can copy and integrate this into your project.
// Browser detection function
const detectBrowser = () => {
const userAgent = navigator.userAgent;
const browsers = [
{ name: 'Brave', test: () => navigator.brave && navigator.brave.isBrave !== undefined, versionRegex: /Chrome\/([0-9.]+)/ },
{ name: 'Yandex Browser', test: /YaBrowser/, versionRegex: /YaBrowser\/([0-9.]+)/ },
{ name: 'Microsoft Edge', test: /Edg/, versionRegex: /Edg\/([0-9.]+)/ },
{ name: 'Mozilla Firefox', test: /Firefox/, versionRegex: /Firefox\/([0-9.]+)/ },
{ name: 'Opera', test: /Opera|OPR/, versionRegex: /(?:Opera|OPR)\/([0-9.]+)/ },
{ name: 'Vivaldi', test: /Vivaldi/, versionRegex: /Vivaldi\/([0-9.]+)/ },
{ name: 'DuckDuckGo Browser', test: /DuckDuckGo/, versionRegex: /DuckDuckGo\/([0-9.]+)/ },
{ name: 'Tor Browser', test: () => userAgent.includes('TorBrowser'), versionRegex: /TorBrowser\/([0-9.]+)/ },
{ name: 'UC Browser', test: /UCBrowser/, versionRegex: /UCBrowser\/([0-9.]+)/ },
{ name: 'Samsung Internet', test: /SamsungBrowser/, versionRegex: /SamsungBrowser\/([0-9.]+)/ },
{ name: 'QQ Browser', test: /QQBrowser/, versionRegex: /QQBrowser\/([0-9.]+)/ },
{ name: 'Baidu Browser', test: /BaiduBrowser/, versionRegex: /BaiduBrowser\/([0-9.]+)/ },
{ name: 'Amazon Silk', test: /Silk/, versionRegex: /Silk\/([0-9.]+)/ },
{ name: 'Vivo Browser', test: /VivoBrowser/, versionRegex: /VivoBrowser\/([0-9.]+)/ },
{ name: 'Miui Browser', test: /MiuiBrowser/, versionRegex: /MiuiBrowser\/([0-9.]+)/ },
{ name: 'Google Chrome', test: /Chrome(?!.*(?:Edg|Brave|YaBrowser))/, versionRegex: /Chrome\/([0-9.]+)/ },
{ name: 'Safari', test: /Safari(?!.*Chrome)/, versionRegex: /Version\/([0-9.]+)/ },
];
// Helper to extract version from userAgent using regex
const getVersion = (regex) => {
const match = userAgent.match(regex);
return match ? match[1] : 'Unknown Version';
};
// Function to detect the operating system
const detectOS = () => {
const osMatchers = [
{ name: 'Windows', regex: /Windows NT ([0-9.]+)/ },
{ name: 'MacOS', regex: /Mac OS X ([0-9._]+)/ },
{ name: 'Linux', regex: /Linux/ },
{ name: 'iOS', regex: /iPhone|iPad/ },
{ name: 'Android', regex: /Android ([0-9.]+)/ },
{ name: 'Android', regex: /Android ([0-9.]+)/ },
];
for (const os of osMatchers) {
const match = navigator.userAgent.match(os.regex);
if (match) {
return { name: os.name, version: match[1] || 'Unknown Version' };
}
}
return { name: 'Unknown OS', version: 'Unknown Version' };
};
// Function to detect the device type
const detectDeviceType = () => {
const userAgent = navigator.userAgent.toLowerCase();
if (/mobi|android|iphone|ipad|windows phone/i.test(userAgent)) return 'mobile';
if (/tablet|ipad/i.test(userAgent)) return 'tablet';
return 'desktop';
};
// Detect other browsers
for (const browser of browsers) {
const isMatch =
typeof browser.test === 'function' ?
browser.test() :
browser.test.test(userAgent);
if (isMatch) {
return {
name: browser.name,
version: getVersion(browser.versionRegex),
os: detectOS(),
deviceType: detectDeviceType(),
};
}
}
// Default fallback
return {
name: 'Unknown Browser',
version: 'Unknown Version',
os: detectOS(),
deviceType: detectDeviceType(),
};
};
Example Usage
// Example usage
const browserInfo = detectBrowser();
console.log(browserInfo);
console.log('Name:', browserInfo.name);
console.log('Version:', browserInfo.version);
console.log('OS name:', browserInfo.os.name);
console.log('OS version:', browserInfo.os.version);
console.log('Device type:', browserInfo.deviceType);
Click the button below and Live preview and edit code your self
Example Output:
{
name: "Google Chrome",
version: "117.0.0",
os: {
name: "Windows",
version: "10.0"
},
deviceType: "Desktop"
}
How It Works
The script uses navigator.userAgent
and regex-based matching to identify browsers and operating systems. It leverages an asynchronous function for Brave browser detection and predefined patterns to determine the user's device type. The final output is a structured JSON object with all the relevant information.
Features of the Browser Detection Script
- Asynchronous detection for Brave browser using
navigator.brave
. - Detects a wide range of browsers including Chrome, Firefox, Edge, Safari, and more.
- Provides detailed operating system information, including version details.
- Identifies the user's device type as mobile, tablet, or desktop.
- Returns consistent and structured data for browser, OS, and device detection.
Live Demo: Preview Your Browser Name And Version Device
- Your browser name : Google Chrome
- Your browser version : 132.0.0.0
- Operating System : Windows
- OS version : 10
- Device Type : desktop
- Brand : unknown
- Has Touch : False
- Is Apple : False
JavaScript Advanced Operating System Detection Code
The operating system detection code identifies the user's OS, such as Windows, MacOS, Linux, iOS, or Android, and extracts its version. By analyzing the user agent string.
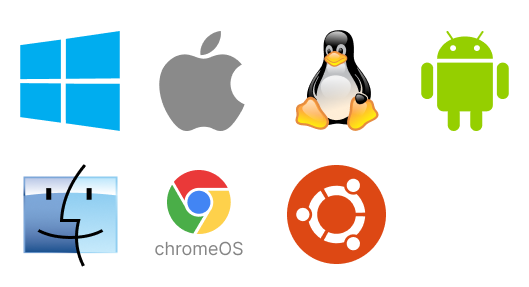
/**
* Detects operating system and version from a user agent string
* @param {string} [userAgent] -
Custom user agent string. If not provided, uses navigator.userAgent
* @returns {Object} Object containing OS name and version
* @throws {Error} If userAgent parameter is provided but not a string
*/
const getOS = (userAgent) => {
// Input validation
if (userAgent !== undefined && typeof userAgent !== 'string') {
throw new Error('userAgent parameter must be a string');
}
// Use provided userAgent or fallback to navigator.userAgent
const ua = userAgent || (typeof navigator !== 'undefined' ? navigator.userAgent : '');
const versionFormatt = version => version.replace(/_/g, '.');
// Enhanced OS detection patterns
const osMatchers = [
{
name: 'Windows',
regex: /Windows NT ([0-9.]+)/,
versionMap: {
'10.0': '10',
'6.3': '8.1',
'6.2': '8',
'6.1': '7',
'6.0': 'Vista',
'5.2': 'Server 2003/XP x64',
'5.1': 'XP',
'5.0': '2000'
}
},
{
name: 'MacOS',
regex: /Mac OS X ([0-9._]+)/,
versionFormatter: versionFormatt
},
{
name: 'iOS',
regex: /OS ([\d._]+) like Mac OS X/,
versionFormatter: versionFormatt
},
{
name: 'iPadOS',
regex: /iPad.*OS ([\d._]+)/,
versionFormatter: versionFormatt
},
{ name: 'Android', regex: /Android ([0-9.]+)/ },
{ name: 'FreeBSD', regex: /FreeBSD/ },
{ name: 'OpenBSD', regex: /OpenBSD/ },
{ name: 'NetBSD', regex: /NetBSD/ },
{ name: 'Solaris', regex: /SunOS/ },
{ name: 'BlackBerry', regex: /BB10|BlackBerry/ },
{ name: 'KaiOS', regex: /KaiOS ([0-9.]+)/ },
{ name: 'Windows Phone', regex: /Windows Phone ([0-9.]+)/ },
{
name: 'Linux',
regex: /Linux/,
distributions: [
{ name: 'Ubuntu', regex: /Ubuntu/ },
{ name: 'Fedora', regex: /Fedora/ },
{ name: 'Red Hat', regex: /Red Hat/ },
{ name: 'Debian', regex: /Debian/ }
]
},
{
name: 'Chrome OS',
regex: /CrOS/
}
];
for (const os of osMatchers) {
const match = ua.match(os.regex);
if (match) {
let version = match[1] || 'Unknown Version';
// Handle Windows version mapping
if (os.name === 'Windows' && os.versionMap?.[version]) {
version = os.versionMap[version];
}
// Handle version formatting (e.g., for MacOS and iOS)
if (os.versionFormatter) {
version = os.versionFormatter(version);
}
// Handle Linux distributions
if (os.name === 'Linux' && os.distributions) {
for (const dist of os.distributions) {
if (ua.match(dist.regex)) {
return {
name: `${dist.name} Linux`,
version: 'Unknown Version',
baseOS: 'Linux'
};
}
}
}
return { name: os.name, version };
}
}
return { name: 'Unknown OS', version: 'Unknown Version' };
};
Example usage
// Example usage:
try {
// Using browser default user agent
const browserOS = getOS();
console.log('name:', browserOS.name); // Windows
console.log('version:', browserOS.version); // 10
// Using a custom user agent string
const customUA = 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_11_2) ... Safari/601.3.9';
const customOS = getOS(customUA);
console.log('Custom OS:', customOS);
// {name: 'MacOS', version: '10.11.2'}
} catch (error) {
console.error('Error detecting OS:', error.message);
}
JavaScript Advanced Device Type Detection Code
The device type detection code determines whether the user is on a mobile, tablet, or desktop device. Using regex-based matching on the user agent string.
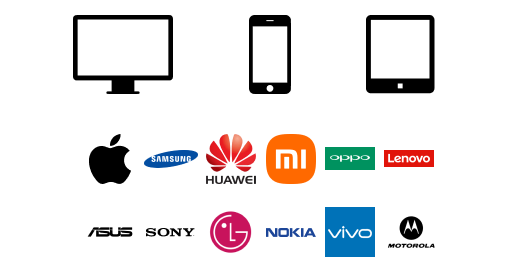
const getDeviceType = () => {
const userAgent = (typeof navigator !== 'undefined' ? navigator.userAgent : '').toLowerCase();
const width = typeof window !== 'undefined' ? window.innerWidth : 0;
const hasTouch = typeof window !== 'undefined' && (
'ontouchstart' in window ||
navigator.maxTouchPoints > 0 ||
navigator.msMaxTouchPoints > 0
);
// Brand patterns
const brandPatterns = {
apple: /(iphone|ipad|ipod|mac)/i,
samsung: /samsung|sm-|galaxy/i,
huawei: /huawei|honor|nova|mate|p\d+/i,
pixel: /pixel|google/i,
xiaomi: /xiaomi|redmi|poco|mi\s|blackshark/i,
surface: /surface/i,
oppo: /oppo|realme|find\sx|reno/i,
vivo: /vivo|iqoo|nex/i,
lenovo: /lenovo/i,
motorola: /motorola|moto\s|razr/i,
asus: /asus|zenfone|rog\sphone/i,
sony: /sony|xperia/i,
lg: /lg|nexus|velvet|wing/i,
nokia: /nokia|hmd/i,
oneplus: /oneplus|nord/i,
zte: /zte|nubia|axon/i,
tcl: /tcl|alcatel/i,
meizu: /meizu|mx\d/i,
blackberry: /blackberry|bb|rim/i,
htc: /htc|desire/i,
nothing: /nothing\sphone/i,
fairphone: /fairphone/i,
cat: /cat phone|caterpillar/i,
sharp: /sharp|aquos/i,
tecno: /tecno|camon|spark/i,
infinix: /infinix|hot|note/i,
essential: /essential\sph/i
};
// Device type patterns
const patterns = {
tablet: /tablet|ipad|playbook|silk|(android(?!.*mobile))/i,
mobile: /android|webos|iphone|ipod|blackberry|iemobile|opera mini|mobile|phone/i,
gamingConsole: /playstation|nintendo|xbox/i,
smartTv: /smart-tv|googletv|netcast|webos|tizen/i,
};
const detectBrand = () => {
for (const [brand, pattern] of Object.entries(brandPatterns)) {
if (pattern.test(userAgent)) return brand;
}
return 'unknown';
};
// Determine device type with additional categories
let deviceType;
if (patterns.tablet.test(userAgent) || (width >= 768 && hasTouch)) {
deviceType = 'tablet';
} else if (patterns.mobile.test(userAgent) || (width < 768 && hasTouch)) {
deviceType = 'mobile';
} else if (patterns.gamingConsole.test(userAgent)) {
deviceType = 'gamingConsole';
} else if (patterns.smartTv.test(userAgent)) {
deviceType = 'smartTv';
} else {
deviceType = 'desktop';
}
return {
type: deviceType,
brand: detectBrand(),
hasTouch,
isApple: brandPatterns.apple.test(userAgent),
};
};
Example usage
// Call the function to detect device details
const deviceInfo = getDeviceType();
// Log the output
console.log("Device Information:", deviceInfo);
console.log("Type:", deviceInfo.type);
console.log("Brand:", deviceInfo.brand);
console.log("Has Touch:", deviceInfo.hasTouch);
console.log("Is Apple:", deviceInfo.isApple);
Example Output Device Type:
On an iPhone:
{
"type": "mobile",
"brand": "apple",
"hasTouch": true,
"isApple": true
}
On a Desktop:
{
"type": "desktop",
"brand": "unknown",
"hasTouch": false,
"isApple": false
}
Features of detectDeviceType
Function
- Detects Device Type: Mobile, Tablet, Desktop, Gaming Console, or Smart TV.
- Recognizes Device Brands: Supports Apple, Samsung, Huawei, Pixel, Xiaomi, Oppo, Vivo, and more.
- Identifies Touch Capability: Checks for touch support using reliable methods.
- Uses Screen Width: Considers screen size for better classification.
- Apple Detection: Flags devices from Apple (iPhone, iPad, Mac).
Notes
- The output varies based on the
userAgent
, screen size, and touch capability of the device. - This example demonstrates flexibility in identifying device types and brands.
JavaScript Function to Identify Brave Browser
This JavaScript code snippet provides an efficient and reliable method to detect if a user is browsing your webpage using the Brave browser. By leveraging built in properties and functions of the navigator
object, the script performs environment checks, ensures compatibility, and gracefully handles errors.
const isBraveBrowser = async () => {
try {
if (navigator.brave && typeof navigator.brave.isBrave === 'function') {
return await navigator.brave.isBrave();
}
return false;
} catch (error) {
console.error('Error checking Brave browser:', error); // Optional logging
return false;
}
};
Example usage
// Usage with then/catch
isBraveBrowser()
.then(isBrave => {
if (isBrave) {
console.log('This is Brave browser');
} else {
console.log('This is not Brave browser');
}
})
.catch(error => {
console.error('Error checking browser:', error);
});
Browser Detection Libraries
While custom JavaScript solutions work well, using specialized libraries can simplify browser detection and handle edge cases more effectively. Here are some popular libraries
1. Bowser
Bowser is a small, fast, and powerful library for detecting browser versions and identifying different mobile or desktop environments. It is highly customizable and frequently updated to support new browser versions.
Usage:
// Install via npm
// npm install bowser
// Example Usage
const Bowser = require('bowser');
const browser = Bowser.getParser(window.navigator.userAgent);
console.log(browser.getBrowserName()); // Outputs browser name
console.log(browser.getBrowserVersion()); // Outputs browser version
console.log(browser.getOSName()); // Outputs operating system name
Features:
- Provides detailed information about the browser, device, and OS.
- Can detect the CPU architecture and device type (mobile, tablet, desktop).
- Frequently updated to recognize new browsers and devices.
When to Use:
When you need a detailed and accurate browser detection with continuous updates for new browsers.
2. UAParser.js
UAParser.js is a lightweight JavaScript library that parses the User-Agent string to extract detailed information about the browser, device, and operating system.
Usage:
// Install via npm
// npm install ua-parser-js
// Example Usage
const UAParser = require('ua-parser-js');
const parser = new UAParser();
const result = parser.getResult();
console.log(result.browser.name); // Outputs browser name
console.log(result.browser.version); // Outputs browser version
console.log(result.device.model); // Outputs device model
Features:
- Provides detailed information about the browser, device, and OS.
- Can detect the CPU architecture and device type (mobile, tablet, desktop).
- Frequently updated to recognize new browsers and devices.
When to Use:
When you need to detect both browser and device information in a lightweight and fast manner.
3. Platform.js
Platform.js is a robust library for detecting operating systems, browsers, engines, and devices. It’s well-known for its accuracy and performance.
Usage
// Install via npm
// npm install platform
// Example Usage
const platform = require('platform');
console.log(platform.name); // Outputs browser name
console.log(platform.version); // Outputs browser version
console.log(platform.os.family); // Outputs OS family
Features:
- Comprehensive detection of browsers, engines, and operating systems.
- Supports a wide range of devices and environments.
- Provides a simple and intuitive API.
When to Use:
When you need detailed platform detection for a wide variety of devices and environments.
4. Detect.js
Detect.js is a small and easy-to-use library for detecting mobile devices and browsers. It is designed for lightweight applications where only essential detection is needed.
Usage
// Install via npm
// npm install detect.js
// Example Usage
const detect = require('detect.js');
const userAgent = detect.parse(navigator.userAgent);
console.log(userAgent.browser.family); // Outputs browser family
console.log(userAgent.device.type); // Outputs device type (mobile, tablet, desktop)
Features:
- Simple and lightweight, focusing on essential detection.
- Supports detecting mobile, tablet, and desktop environments.
- Can identify popular browser families and versions.
When to Use:
When you need a straightforward solution for detecting mobile browsers or basic browser identification.
5. Browser-Detect
Browser-Detect is a minimalistic library for browser and OS detection. It focuses on providing the most common and necessary information about the user's browser and platform.
Usage
// Install via npm
// npm install browser-detect
// Example Usage
const browser = require('browser-detect');
const result = browser();
console.log(result.name); // Outputs browser name
console.log(result.version); // Outputs browser version
console.log(result.os); // Outputs operating system name
Features:
- Simple API for detecting browsers, versions, and operating systems.
- Lightweight and easy to integrate into existing projects.
- Covers the most commonly used browsers.
When to Use:
When you need quick and basic browser detection with minimal setup.
6. FingerprintJS
FingerprintJS is a powerful library that not only detects the browser but also creates a unique fingerprint for each visitor, which can be used for identification purposes.
Usage
// Install via npm
// npm install @fingerprintjs/fingerprintjs
// Example Usage
const FingerprintJS = require('@fingerprintjs/fingerprintjs');
FingerprintJS.load().then(fp => fp.get()).then(result => {
console.log(result.visitorId); // Outputs unique visitor ID
console.log(result.components.userAgent.browser.family); // Outputs browser family
});
Features:
- Generates a unique fingerprint for each visitor.
- Provides detailed information about the browser and device.
- Useful for fraud prevention, analytics, and security.
When to Use:
When you need to track users uniquely across different sessions or detect bots and fraud attempts.
These libraries are especially useful when you need to support a broad range of devices and browsers.