How to Create JavaScript Arrays and Objects with Alphabet and Numbers
You are working with characters or numbers, knowing how to quickly generate arrays and objects can save you valuable time.
In this article, we will explore various methods to create JavaScript arrays containing the alphabet (a-z) and numeric values (0-9), as well as how to build corresponding objects. We will cover everything from basic loops to more advanced techniques using Array.from, reduce, and other handy methods.
Create a shortcut JavaScript array containing the letters 'a' through 'z', you can use various methods. Here are a few options.
Creating an Array of Alphabet Letters (a-z)
Simple and Efficient Ways to Generate Alphabet Arrays
Creating an array containing the letters 'a' through 'z' is a common task in JavaScript. There are several ways to achieve this, each with its own benefits. Below are a few methods that range from using traditional loops to leveraging more modern JavaScript features.
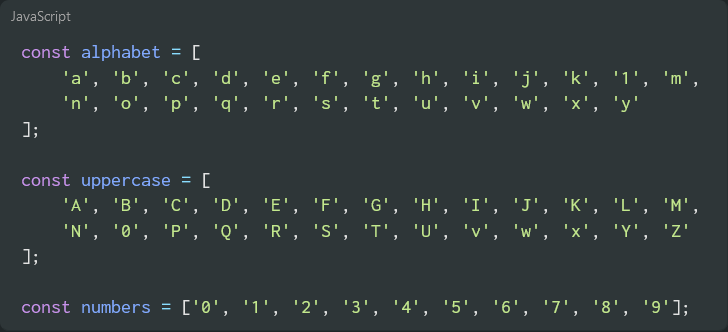
Lowercase Alphabet Array
Code Example 1
Using Array.from
and String.fromCharCode.
This approach uses Array.from
to create an array of 26 elements, each corresponding to a letter in the alphabet, starting from 'a' (ASCII code 97). It is a clean and concise method.
const alphabet = Array.from({ length: 26 }, (_element, index) => String.fromCharCode(97 + index));
console.log(alphabet);
// output
// ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
Click the button below and Live preview and edit code your self
Try it YourselfUppercase Alphabet Array
const uppercaseAlphabet = Array.from({ length: 26 }, (_element, index) => String.fromCharCode(65 + index));
console.log(uppercaseAlphabet);
// output
// ['A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P','Q','R','S','T','U','V','W','X','Y','Z']
Code Example 2
Using a for
Loop.
The for
loop method is straightforward and easy to understand, especially for beginners. It iterates through the ASCII codes for 'a' to 'z' and pushes each character into the array.
const alphabet = [];
for (let i = 97; i <= 122; i++) {
alphabet.push(String.fromCharCode(i));
}
console.log(alphabet);
// output
// ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
Uppercase
const alphabetUppercase = [];
for (let i = 65; i <= 90; i++) {
alphabetUppercase.push(String.fromCharCode(i));
}
console.log(alphabetUppercase);
// output
// ['A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P','Q','R','S','T','U','V','W','X','Y','Z']
Creating an Array of Numbers (0-9)
Generating Numeric Arrays in JavaScript
Generating an array containing the numbers 0 through 9 is just as simple as creating an alphabet array. Here are a few methods to create a numeric array, with each element represented as a string.
Code Example 1
Using Array.from
and toString()
.
This method leverages Array.from
to create an array of numbers from 0 to 9, converting each number to a string using toString()
. It is a clean and efficient solution.
const numbers = Array.from({ length: 10 }, (_element, index) => index.toString());
console.log(numbers);
// output ['0','1','2','3','4','5','6','7','8','9']
Code Example 2
Using a for
Loop.
The for loop is a classic way to generate arrays in JavaScript. It iterates over each number from 0 to 9, converting each to a string and adding it to the array.
const numbers = [];
for (let i = 0; i <= 9; i++) {
numbers.push(i.toString());
}
console.log(numbers);
// output ['0','1','2','3','4','5','6','7','8','9']
Creating Objects with Alphabet and Numbers
In some cases, you might need to create objects where each key is a letter or number, and the value is its string representation. This is particularly useful in scenarios where you need to quickly look up values based on keys.
Numbers Object
Using for Loop to Create Number Object
const numbersObject = {};
for (let i = 0; i <= 9; i++) {
numbersObject[i] = i.toString();
}
console.log(numbersObject);
// output object
// { '0': '0', '1': '1', '2': '2', ... '9': '9' }
Using Object.fromEntries
const numbersObject = Object.fromEntries(
Array.from({ length: 10 }, (_element, index) => [index, index.toString()])
);
console.log(numbersObject);
// output object
// { '0': '0', '1': '1', '2': '2', ... '9': '9' }
Alphabet Objects
Creating an object with alphabet letters as both the keys and values is a common requirement, especially when dealing with letter-based lookup or character mappings. Here are a few efficient ways to generate such objects in JavaScript.
Alphabet lowercase object
Object.fromEntries
takes an array of key-value pairs and turns it into an object. This approach is modern and efficient, especially when you need to create objects dynamically.
const alphabetLowercaseObject = Object.fromEntries(
Array.from({ length: 26 }, (_element, index) => {
const letter = String.fromCharCode(97 + index);
return [letter, letter];
})
);
console.log(alphabetLowercaseObject);
// output object
// { 'a': 'a', 'b': 'b', 'c': 'c', ... 'z': 'z' }
Alphabet uppercase object
const alphabetUppercaseObject = Object.fromEntries(
Array.from({ length: 26 }, (_element, index) => {
const letter = String.fromCharCode(65 + index);
return [letter, letter];
})
);
console.log(alphabetUppercaseObject);
// output object
// { 'A': 'A', 'B': 'B', 'C': 'C', ... 'Z': 'Z' }
Alphabet and Number Array Use Cases
- Quizzes and Games: Generate alphabet arrays to create quizzes or games that test users' knowledge of the alphabet, such as guessing letters or word scrambles.
- Random Word Generation: Use alphabet arrays to generate random words for word games, puzzles, or creative writing prompts.
- Data Visualization: Utilize number arrays to create data visualizations, such as charts or graphs, to represent trends or patterns.
- Password Generation: Create strong passwords by combining alphabet and number arrays, ensuring a mix of characters and numbers.
- Lottery Number Generation: Generate random numbers for lottery tickets or raffles.
- Alphabetical Sorting: Use alphabet arrays to sort lists of words or names in alphabetical order.
- Number Sequences: Generate number arrays for mathematical sequences, such as Fibonacci or prime numbers.
- Educational Tools: Create interactive educational tools, like alphabet or number matching games, to help students learn and practice.